SEPA Integration
Introduction
The operation is detailed for sending PAYCOMET the data of operations to be carried out under the SEPA framework, as well as the documentation required for the recipients of these operations, through the webservices established for this purpose.
The communication will be carried out through SSL protocol, actively rejecting any unsecured delivery of information.
The following data will be provided by PAYCOMET for proper integration:
- Supplier ID.
- Supplier PASSWORD (for securing signatures).
In most cases, it's recommended to start integrating the SEPA documentation section, as uploading documents is the first thing that must be done. After that, you can integrate the operations procedure.
If the system is not able to verify the uploaded documentation, the verification process can take up to 24 or 48 hours.
SEPA flow chart
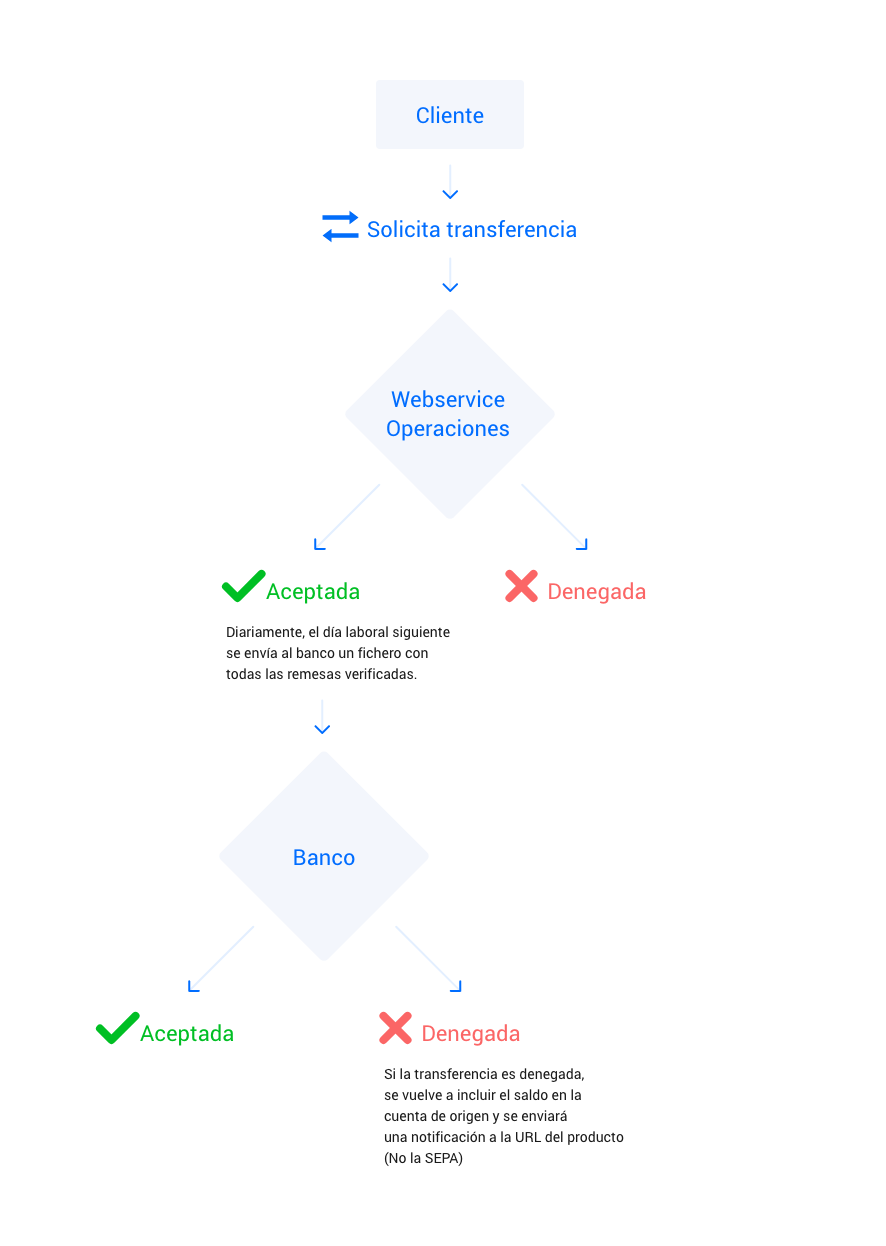
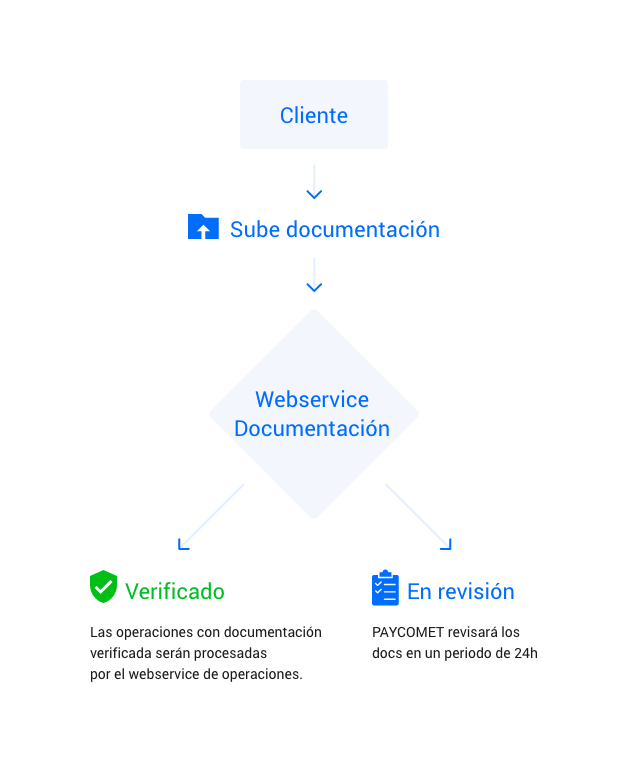
SEPA operations
El acceso al servicio para la carga de operaciones está disponible en las integraciones REST y XML.
Important
XML integration is deprecated. We recommend using REST integration.
Method sepaOperations
The call to this method will allow the delivery of the information associated with the SEPA operations: Direct Debits or Transfers, between products of the same account. All fields will be declared as string and are obligatory; if in any operation one is lacking information, it must be sent as an empty string.
Select the type of integration:
The details on this functions are explained in REST full documentation, available in this link
https://api.paycomet.com/gateway/xml-sepa?wsdl
Input parameters:
Element | Content | Description |
---|---|---|
providerID | Obligatory. The unique identifier assigned by PAYCOMET for the supplier sending SEPA operations. Available on the client control panel. | |
operations | Array | Obligatory. Array of operations reported. |
The array of operations must have the following format:
Element | Content | Description |
---|---|---|
operationType | Types of operations: 1 – Direct Debit (N19) 2 – Transfer (N34) |
Obligatory. Type of operation. Identifies whether the operation is a direct debit or a transfer. |
merchantCode | [A-Za-z0-9]{1,8} | Obligatory. Client code. Obtained from the control panel. |
terminalIDDebtor | [0-9]{1,5} | Obligatory. This will be the terminal number assigned to the product. Obtained from the control panel. Identifies the terminal number of the debtor/payer of a SEPA operation. Therefore, it will depend on the type of operation (debit, transfer). |
uniqueIdCreditor | [a-zA-Z0-9] | Obligatory. This will be the unique identifier of this individual, freelancer, company (creditor/recipient) in the client. |
companyNameCreditor | [a-zA-Z0-9] | Obligatory. Name of the company, individual or freelancer corresponding to the previous indicator. |
ibanNumberCreditor | [a-zA-Z0-9] | Obligatory. IBAN code of the account of the creditor/recipient. |
swiftCodeCreditor | [a-zA-Z0-9] | SWIFT code of the bank account of the creditor/recipient. Must be provided when the account IBAN is not Spanish. If the ibanNumberCreditor number belongs to a Spanish account, it must be sent as an empty string. |
companyTypeCreditor | [0-9]{1,3} | Obligatory. Identifier of the type of creditor/recipient: 1: Individual / 2: Freelancer / 3: Commercial Company. |
operationOrder | [a-zA-Z0-9] | Unique reference of the operation. |
operationAmount | [0-9]{1,8} | Obligatory. Amount in the transaction currency with 2 decimals in integer format: (€2.25 = 225). |
operationCurrency | [EUR][USD][GBP][JPY] | Obligatory. Currency type of the transaction. The only permitted currency is the euro, whose code is "EUR". |
operationDatetime | [0-9]{yyyymmdd} | Obligatory. Date desired for sending the SEPA operation / remittance. Always after the current date. Format: yyyymmdd. |
operationConcept | [A-Za-z0-9]|[+|?|\/|-|:|(|)|\.|,|'| ]{100} | Obligatory. Concept assigned to the operation / remittance. This is the descriptor which will appear in banking entries. Maximum length 100. Although error 1273 specified 140 characters, PAYCOMET reserves 40, the maximum permitted in the input therefore being 100. |
operationSignature | [a-zA-Z0-9] | Obligatory. Signature to check the validity of the operation. Will be calculated in accordance with CALCULATION OF THE SIGNATURE. |
Response parameters:
Element | Content | Description |
---|---|---|
operationResult | OK|KO | Operation result. |
operationErrorCode | [0-9]{1-4} | In case of operationResult KO, the error ID obtained is returned. See ERROR CODES. |
operationOrder | [a-zA-Z0-9] | Unique reference of the operation. |
array (size=2)
0 => object(sepaOperationResponseClass)[7]
public 'operationResult' => string 'OK' (length=2)
public 'operationErrorCode' => string '' (length=0)
public 'operationOrder' => string 'AV-PROD-TEST-9987023' (length=20)
1 => object(sepaOperationResponseClass)[30]
public 'operationResult' => string 'KO' (length=2)
public 'operationErrorCode' => string '1026' (length=4)
public 'operationOrder' => string 'AV-PROD-TEST-9987023' (length=20)
Signature calculation
The calculation of the signature for each of the operations to be reported will be carried out as follows:
In pseudocode:
plainSignature = operationOrder + operationType + operationAmount + operationCurrency + providerID + merchantCode + terminalIDDebtor + uniqueIdCreditor + ibanNumberCreditor + swiftCodeCreditor + companyTypeCreditor + PASSWORD
finalSignature = TOUPPER(SHA512(plainSignature))
In PHP code:
<?php
$plainSignature = ($operation->operationOrder . $operation->operationType . $operation->operationAmount . $operation->operationCurrency . $providerID . $merchantCode . $operation->terminalIDDebtor . $operation->uniqueIdCreditor . $operation->ibanNumberCreditor . $operation->swifCodeCreditor . $operation->companyTypeCreditor . $providerPassword);
$signature = strtoupper(hash('sha512', $plainSignature));
SEPA operations insertion example
<?php
/**
* SEPA operations insertion example
* Documentation and specificacions: https://docs.paycomet.com/en/documentacion/sepa
*
* Tracking ID: BGD-2G6-2BB6
*
* @author PAYTPV ONLINE ENTIDAD DE PAGO, S.L.
* @copyright Copyright (c) 2019, PAYTPV ONLINE ENTIDAD DE PAGO, S.L.
* @version 1.0 2016-01-28
*/
date_default_timezone_set("Europe/Madrid");
$timeStart = microtime(true);
// Si se utiliza xdebug
ini_set("xdebug.var_display_max_data",-1);
ini_set("xdebug.var_display_max_children",-1);
ini_set("xdebug.var_display_max_depth",-1);
ini_set("soap.wsdl_cache_enabled", 0);
ini_set("soap.wsdl_cache_ttl", 0);
ini_set("soap.wsdl_cache_limit",0);
class sepaOperations {
public $providerID = 0;
public $operationsArray = array();
}
class operation {
public $operationType;
public $merchantCode;
public $terminalIDDebtor;
public $terminalIDCreditor;
public $operationOrder;
public $operationAmount;
public $operationCurrency;
public $operationDatetime;
public $operationConcept;
public $operationSignature;
}
$endPoint = "https://api.paycomet.com/gateway/xml-sepa?wsdl";
$providerID = "incluirProviderID";
$password = "incluirProviderPassword";
$client = new SOAPClient($endPoint);
$numOperations = 1; // Example for a single operation
//-------------------------------------------------------------- sepaOperations ------------------------------------------------------------------->
$arrOperations = array();
for ($n=1;$n<=$numOperations;$n++) {
$operation = new operation();
$operation->operationType = "2"; // 2: Transfer (N34)
$operation->merchantCode = "incluirMerchantCode"; // Merchant code. Available in control panel.
$operation->terminalIDDebtor = "incluirTerminalIdDelDeudor"; // Terminal number assigned to the product. Available in control panel.
$operation->uniqueIdCreditor = "incluirIdentificadorUnicoDeClienteEnVuestroSistema"; // Unique identifier for that individual, freelance or company in your system
$operation->companyNameCreditor = "incluirNombreDeCliente"; // Individual, freelance or company name, coresponding to the last identifier
$operation->ibanNumberCreditor = "incluirIBANValido"; // IBAN code of the destination account
$operation->swiftCodeCreditor = ""; // SWIFT code of the destination account. Recommended not spanish IBAN accounts
$operation->companyTypeCreditor = 3; // 1: Individual, 2: Freelance, 3: Company
$operation->operationOrder = "SEPA TRANSFER-".date("Ymd H:i:s") . substr((string)microtime(), 1, 8); // Operation unique reference
$operation->operationAmount = "1000"; // Amount in the transaction currency, including 2 decimals in integer format: (2,25 € = 225).
$operation->operationCurrency = "EUR"; // Transaction currency. Only EUR is allowed
$operation->operationDatetime = "20190712"; // yyyyymm, date on which the operation will be sent / SEPA batch. Always greater than today
$operation->operationConcept = "Transfer XXXXXXXXX - to XXXXXXXXX: ".rand(0,9999); // Description to be shown in the bank statement. Max length 100 characters
// Signature calculation
$plainSignature = ($operation->operationOrder.$operation->operationType.$operation->operationAmount.$operation->operationCurrency.$providerID.$operation->merchantCode.$operation->terminalIDDebtor.$operation->uniqueIdCreditor.$operation->ibanNumberCreditor.$operation->swiftCodeCreditor.$operation->companyTypeCreditor.$password);
$signature = strtoupper(hash("sha512" ,$plainSignature));
$operation->operationSignature = $signature;
// Because single element arrays don't assign numeric $key.
$arrOperations[$n] = $operation;
}
//--------------------------------------------------------------->
$postData = new sepaOperations();
$postData->providerID = $providerID;
$postData->operationsArray = $arrOperations;
var_dump($arrOperations);
$timeRequest = microtime(true);
$response = $client->sepaOperations($postData->providerID, $postData->operationsArray);
$timeResponse = microtime(true);
var_dump($response);
$time = $timeResponse - $timeRequest;
echo "
Requests number: $numOperations - Response in $time";
$timeEnd = microtime(true);
?>
Method sepaCancel
Calling this method will allow you to cancel a SEPA transaction that has not been settled at the merchant
Select the type of integration:
The details on this functions are explained in REST full documentation, available in this link
https://api.paycomet.com/gateway/xml-sepa?wsdl
Input parameters:
Element | Content | Description |
---|---|---|
providerID | Obligatory. The unique identifier assigned by PAYCOMET for the supplier sending SEPA operations. Available on the client control panel. | |
merchantCode | [A-Za-z0-9]{1,8} | Obligatory. Client code. Obtained from the control panel. |
terminalIDDebtor | [0-9]{1,5} | Obligatory. This will be the terminal number assigned to the product. Obtained from the control panel. Identifies the terminal number of the debtor/payer of a SEPA operation. Therefore, it will depend on the type of operation (debit, transfer). |
operationOrder | [a-zA-Z0-9] | Unique reference of the operation. |
operationSignature | [a-zA-Z0-9] | Obligatory. Signature to check the validity of the operation. Will be calculated in accordance with CALCULATION OF THE SIGNATURE. |
Response parameters:
Element | Content | Description |
---|---|---|
operationResult | OK|KO | Operation result. |
operationErrorCode | [0-9]{1-4} | In case of operationResult KO, the error ID obtained is returned. See ERROR CODES. |
operationOrder | [a-zA-Z0-9] | Unique reference of the operation. |
Signature calculation
The calculation of the signature for each of the operations to be reported will be carried out as follows:
In pseudocode:
plainSignature = operationOrder + providerID + merchantCode + terminalIDDebtor + PASSWORD
finalSignature = TOUPPER(SHA512(plainSignature))
In PHP code:
<?php
$plainSignature = ($operation->operationOrder . $providerID . $merchantCode . $operation->terminalIDDebtor . $providerPassword)
SEPA documentation
Access to the service can be made through XML and REST integrations.
Method add_document
The call to this method will allow the sending of the documentation requested from clients who will make use of the SEPA operations service. All fields are obligatory.
Select the type of integration:
The details on this functions are explained in REST full documentation, available in this link
https://api.paycomet.com/gateway/xml-sepa-documentation?wsdl
Input parameters:
Element | Content | Description |
---|---|---|
SEPA_PROVIDER_ID | [A-Za-z0-9] | Uniquue identifier assigned by PAYCOMET for the supplier sending SEPA operations. Available on the client control panel. |
MERCHANT_CODE | [A-Za-z0-9]{1,8} | Unique identifier as PAYCOMET account. Available on the client control panel. |
MERCHANT_CUSTOMER_ID | [A-Za-z0-9] | Unique identifier of the client of the supplier. |
MERCHANT_CUSTOMER_IBAN | [a-zA-Z0-9] | Account number of the client in IBAN format. |
DOCUMENT_TYPE | [0-9] | Identifier of the type of document on PAYCOMET. See TYPES OF DOCUMENT. |
FILE_CONTENT | [A-Za-z0-9] | Binary content of the file to send, codified in base 64. |
SIGNATURE | [A-Za-z0-9] | Signature of the request. See CALCULATION OF THE SIGNATURE. |
NOTE: The maximum size of the document is limited to 8Mb. The permitted document types are: PDF, DOC, DOCX, ODT, JPG and PNG.
Response parameters:
Element | Content | Description |
---|---|---|
MERCHANT_CUSTOMER_ID | [A-Za-z0-9] | Unique identifier of the client of the supplier. |
MERCHANT_CUSTOMER_IBAN | [A-Za-z0-9] | Account number of the client in IBAN format. |
DOCUMENT_TYPE | [0-9] | Identifier of the type of document on PAYCOMET. See TYPES OF DOCUMENT. |
DOCUMENT_STATUS | [0-9] | Identifier of the document status. See DOCUMENT STATUSES |
ERROR_ID | [0-9] | In case of error, the error identifier obtained is returned. See ERROR CODES. |
Signature calculation add_document
The calculation of the signature for the request will be carried out as follows:
SHA512( SEPA_PROVIDER_ID + MERCHANT_CODE + MERCHANT_CUSTOMER_ID + MERCHANT_CUSTOMER_IBAN + DOCUMENT_TYPE + PROVIDER_PASSWORD )
PHP
<?php
$data['SIGNATURE'] = hash('sha512', $data['SEPA_PROVIDER_ID'] . $data['MERCHANT_CODE'] . $data['MERCHANT_CUSTOMER_ID'] . $data['MERCHANT_CUSTOMER_IBAN'] . $data['DOCUMENT_TYPE'] . $providerPassword);
Method check_document
The call to this method will allow the status of a document sent to PAYCOMET to be consulted for verification. All fields are obligatory.
Select the type of integration:
The details on this functions are explained in REST full documentation, available in this link
https://api.paycomet.com/gateway/xml-sepa-documentation?wsdl
Input parameters:
Element | Content | Description |
---|---|---|
SEPA_PROVIDER_ID | [A-Za-z0-9] | Uniquue identifier assigned by PAYCOMET for the supplier sending SEPA operations. Available on the client control panel. |
MERCHANT_CODE | [A-Za-z0-9]{1,8} | Unique identifier as PAYCOMET account. Available on the client control panel. |
MERCHANT_CUSTOMER_ID | [A-Za-z0-9] | Unique identifier of the client of the supplier. |
MERCHANT_CUSTOMER_IBAN | [A-Za-z0-9] | Account number of the client in IBAN format. |
DOCUMENT_TYPE | [0-9] | Identifier of the type of document on PAYCOMET. See TYPES OF DOCUMENT. |
SIGNATURE | [A-Za-z0-9] | Signature of the request. See CALCULATION OF THE SIGNATURE. |
Response parameters:
Element | Content | Description |
---|---|---|
MERCHANT_CUSTOMER_ID | [A-Za-z0-9] | Unique identifier of the client of the supplier. |
MERCHANT_CUSTOMER_IBAN | [A-Za-z0-9] | Account number of the client in IBAN format. |
DOCUMENT_TYPE | [0-9] | Identifier of the type of document on PAYCOMET. See TYPES OF DOCUMENT. |
DOCUMENT_STATUS | [0-9] | Identifier of the document status. See DOCUMENT STATUSES |
ERROR_ID | [0-9] | In case of error, the error identifier obtained is returned. See ERROR CODES. |
Signature calculation check_document
The calculation of the signature for the request will be carried out as follows:
SHA512( SEPA_PROVIDER_ID + MERCHANT_CODE + MERCHANT_CUSTOMER_ID + MERCHANT_CUSTOMER_IBAN + DOCUMENT_TYPE + PROVIDER_PASSWORD )
PHP
<?php
$data['SIGNATURE'] = hash('sha512', $data['SEPA_PROVIDER_ID'] . $data['MERCHANT_CODE'] . $data['MERCHANT_CUSTOMER_ID'] . $data['MERCHANT_CUSTOMER_IBAN'] . $data['DOCUMENT_TYPE'] . $providerPassword);
Method check_customer
The call to this method will check the status of the documentation sent to PAYCOMET to be consulted for verification. All fields are obligatory.
Select the type of integration:
The details on this functions are explained in REST full documentation, available in this link
https://api.paycomet.com/gateway/xml-sepa-documentation?wsdl
Input parameters:
Element | Content | Description |
---|---|---|
SEPA_PROVIDER_ID | [A-Za-z0-9] | Uniquue identifier assigned by PAYCOMET for the supplier sending SEPA operations. Available on the client control panel. |
MERCHANT_CODE | [A-Za-z0-9]{1,8} | Unique identifier as PAYCOMET account. Available on the client control panel. |
MERCHANT_CUSTOMER_ID | [A-Za-z0-9] | Unique identifier of the client of the supplier. |
MERCHANT_CUSTOMER_IBAN | [A-Za-z0-9] | Account number of the client in IBAN format. |
MERCHANT_CUSTOMER_TYPE | [0-9] | Identifier of the type of creditor/recipient: 1: Individual / 2: Freelancer / 3: Commercial Company. |
SIGNATURE | [A-Za-z0-9] | Signature of the request. See CALCULATION OF THE SIGNATURE. |
Response parameters:
Element | Content | Description |
---|---|---|
MERCHANT_CUSTOMER_ID | [A-Za-z0-9] | Unique identifier of the client of the supplier. |
MERCHANT_CUSTOMER_IBAN | [A-Za-z0-9] | Account number of the client in IBAN format. |
DOCUMENTS | [A-Za-z0-9] | Text string in JSON format with the information of the documents status. The information contains the document type identifier and a boolean value to identify if it's verified or not. See TYPES OF DOCUMENT. |
ERROR_ID | [0-9] | In case of error, the error identifier obtained is returned. See ERROR CODES. |
Signature calculation check_customer
The calculation of the signature for the request will be carried out as follows:
SHA512( SEPA_PROVIDER_ID + MERCHANT_CODE + MERCHANT_CUSTOMER_ID + MERCHANT_CUSTOMER_IBAN + PROVIDER_PASSWORD )
PHP
<?php
$data['SIGNATURE'] = hash('sha512', $data['SEPA_PROVIDER_ID'] . $data['MERCHANT_CODE'] . $data['MERCHANT_CUSTOMER_ID'] . $data['MERCHANT_CUSTOMER_IBAN'] . $providerPassword);
SEPA documents upload and check example
<?php
/**
* Test para añadir y comprobar documentos SEPA
* Documentación y especificaciones: https://docs.paycomet.com/en/documentacion/sepa
*
* @author PAYCOMET
* @copyright Copyright (c) 2020, PAYCOMET
*/
date_default_timezone_set("Europe/Madrid");
ini_set("soap.wsdl_cache_enabled", "0");
ini_set("soap.wsdl_cache_ttl", "0");
$endpoint = "https://api.paycomet.com/gateway/xml-sepa-documentation?wsdl";
$context = stream_context_create([
"ssl" => [
"verify_peer" => false,
"verify_peer_name" => false,
"allow_self_signed" => true
]
]);
$options = [
"use" => "literal",
"stream_context" => $context
];
########################
##### add_document #####
########################
$fileContent = base64_encode(
file_get_contents("path_to_file.pdf")
);
$params = [];
$params["SEPA_PROVIDER_ID"] = "*** YOUR PROVIDER ID ***";
$params["MERCHANT_CODE"] = "*** YOUR MERCHANT CODE ***";
$params["MERCHANT_CUSTOMER_ID"] = "*** YOUR CUSTOMER ID";
$params["MERCHANT_CUSTOMER_IBAN"] = "ES*******************";
$params["DOCUMENT_TYPE"] = "*** YOUR DOCUMENT TYPE ID ***";
$params["FILE_CONTENT"] = $fileContent;
$providerPassword = "*** YOUR PROVIDER PASSWORD ***";
$params["SIGNATURE"] = hash("sha256", $params["SEPA_PROVIDER_ID"] . $params["MERCHANT_CODE"] . $params["MERCHANT_CUSTOMER_ID"] . $params["MERCHANT_CUSTOMER_IBAN"] . $params["DOCUMENT_TYPE"] . $providerPassword);
$client = new SoapClient($endpoint, $options);
$response = $client->add_document($params["SEPA_PROVIDER_ID"], $params["MERCHANT_CODE"], $params["MERCHANT_CUSTOMER_ID"], $params["MERCHANT_CUSTOMER_IBAN"], $params["DOCUMENT_TYPE"], $params["FILE_CONTENT"], $params["SIGNATURE"]);
var_dump($response);
die();
##########################
##### check_document #####
##########################
$params = [];
$params["SEPA_PROVIDER_ID"] = "*** YOUR PROVIDER ID ***";
$params["MERCHANT_CODE"] = "*** YOUR MERCHANT CODE ***";
$params["MERCHANT_CUSTOMER_ID"] = "*** YOUR CUSTOMER ID";
$params["MERCHANT_CUSTOMER_IBAN"] = "ES*******************";
$params["DOCUMENT_TYPE"] = "*** YOUR DOCUMENT TYPE ID ***";
$providerPassword = "*** YOUR PROVIDER PASSWORD ***";
$params["SIGNATURE"] = hash("sha256", $params["SEPA_PROVIDER_ID"] . $params["MERCHANT_CODE"] . $params["MERCHANT_CUSTOMER_ID"] . $params["MERCHANT_CUSTOMER_IBAN"] . $params["DOCUMENT_TYPE"] . $providerPassword);
$client = new SoapClient($endpoint, $options);
$response = $client->check_document($params["SEPA_PROVIDER_ID"], $params["MERCHANT_CODE"], $params["MERCHANT_CUSTOMER_ID"], $params["MERCHANT_CUSTOMER_IBAN"], $params["DOCUMENT_TYPE"], $params["SIGNATURE"]);
var_dump($response);
die();
Method del-customer-iban
The call to this method deletes the documentation of a registered IBAN.
IMPORTANT!! Once this is done, if you want to use the IBAN again, you would have to upload all your documentation again.
The details on this functions are explained in REST full documentation, available in this link
Method enrole_customer
Enrole customer allows the merchant to obtain a link where the customer may complete some of the required document (Certification of account ownership at the moment) identifies theme selves in his bank account.
The details on this functions are explained in REST full documentation, available in this link
Appendixes
Appendix I - Types of document
DOCUMENT_TYPE | Description | Enrolment link available? |
---|---|---|
1 | DNI identity document/PASSPORT (Front) True holder | |
2 | DNI identity document/PASSPORT (Reverse) True holder | |
3 | Certification of account ownership | Avalaible |
4 | Bank receipt | |
5 | Freelancer receipt or form 36/37 | |
6 | DNI identity document/PASSPORT (Front) > 25% | |
7 | DNI identity document/PASSPORT (Reverse) > 25% | |
8 | DNI identity document/PASSPORT (Front) Administrator | |
9 | DNI identity document/PASSPORT (Reverse) Administrator | |
10 | Power of attorney | |
11 | Articles of Incorporation and other pertinent deeds | |
12 | CIF tax identification number | |
13 | Responsible declaration of validity of the documentation | |
14 | Certification of being up to date with tax obligations | |
15 | Activity licences | |
16 | PAYCOMET contract | |
17 | SEPA document | |
18 | NON-SEC operation declaration | |
19 | Supplementary licence form | |
20 | Invoice or receipt accrediting the services provided | |
21 | Last declaration of IRPF personal income tax and VAT | |
22 | Financial statements, last declaration of VAT and corporation tax | |
23 | Tax identification card | |
24 | Deeds of powers |
Appendix II - Document statuses
DOCUMENT_STATUS | Description |
---|---|
2 | Under review |
3 | Verified |